Dynamic Title for Webpage in Vue JS
Creating a dynamic title for a webpage in Vue.js is a common requirement, especially for enhancing SEO and providing a better user experience. Here’s a step-by-step guide on how to achieve this from scratch.
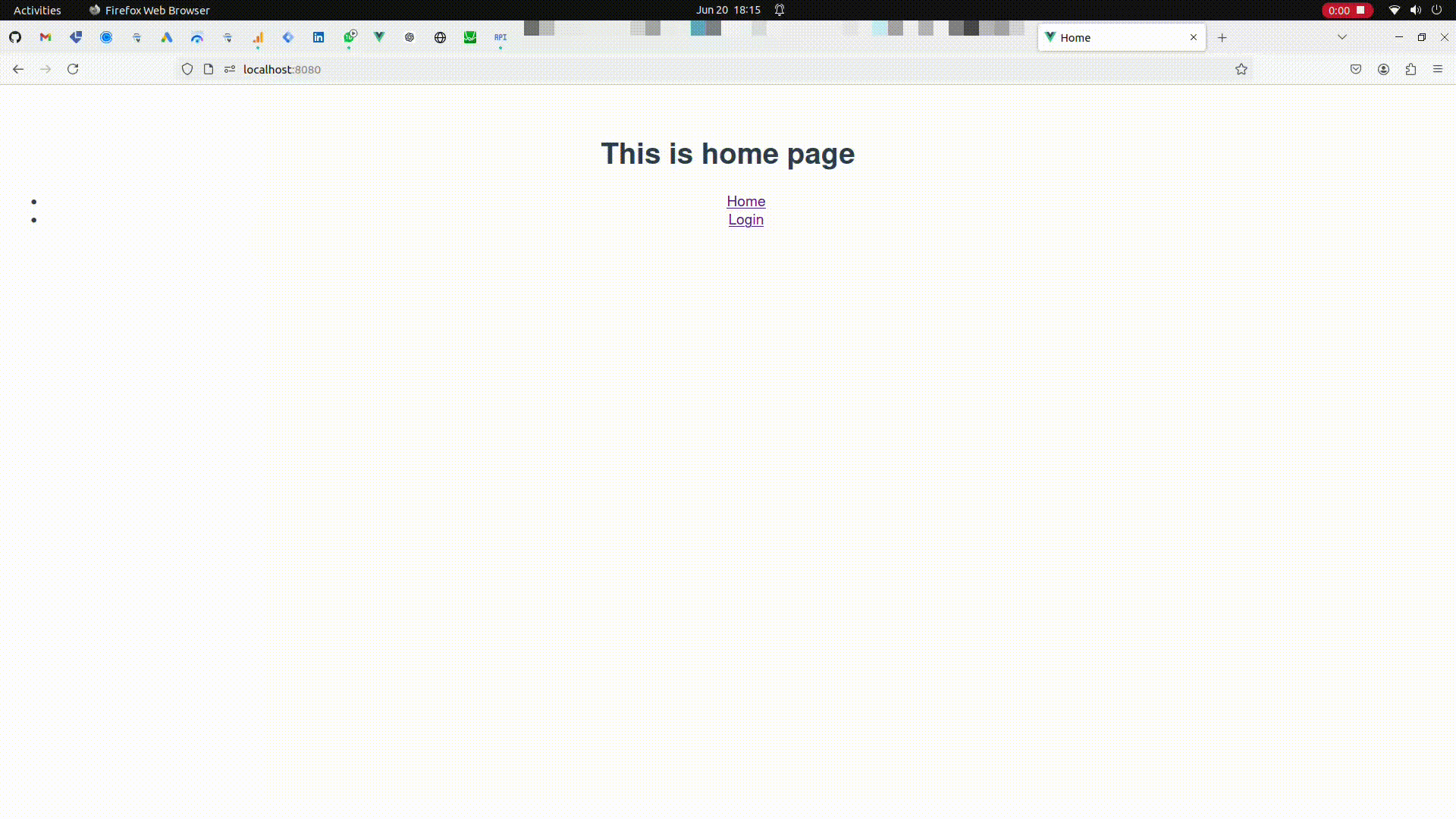
Step 1: Set Up Your Vue Project
First, ensure you have Vue CLI installed. If not, you can install it globally using npm:
npm install -g @vue/cli
Create a new Vue project:
vue create dynamic-title-demo
Navigate to your project directory:
cd dynamic-title-demo
Step 2: Set Up Routes with Meta Titles and Navigation Guard
In your router/index.js
, define routes, add meta titles, and include the beforeEach
navigation guard:
import Vue from ‘vue’;
import Router from ‘vue-router’;
import Home from ‘@/components/Home.vue’;
import About from ‘@/components/About.vue’;
Vue.use(Router);
const router = new Router({
routes: [
{
path: ‘/’,
name: ‘Home’,
component: Home,
meta: { title: ‘Home Page’ },
},
{
path: ‘/about’,
name: ‘About’,
component: About,
meta: { title: ‘About Us’ },
},
],
});
router.beforeEach((to, from, next) => {
document.title = to.meta.title || ‘Default Title’;
next();
});
export default router;
Step 3: Modify main.js
In your main.js
file, simply import and use the router without needing to add the navigation guard:
import Vue from ‘vue’;
import App from ‘./App.vue’;
import router from ‘./router’;
Vue.config.productionTip = false;
new Vue({
router,
render: h => h(App),
}).$mount(‘#app’);
Step 4: Create Sample Components
Create the Home.vue
and About.vue
components inside the src/components
directory.
Home.vue
<template>
<div>
<h1>Home Page</h1>
<p>Welcome to the Home Page.</p>
</div>
</template>
<script>
export default {
name: ‘Home’,
};
</script>
<style scoped>
/* Your styles here */
</style>
About.vue
<template>
<div>
<h1>About Us</h1>
<p>Learn more about us on this page.</p>
</div>
</template>
<script>
export default {
name: ‘About’,
};
</script>
<style scoped>
/* Your styles here */
</style>
Step 5: Update App.vue
Ensure your App.vue
has the <router-view>
to display your components based on the route:
<template>
<div id=”app”>
<router-view></router-view>
</div>
</template>
<script>
export default {
name: ‘App’,
};
</script>
<style>
/* Your styles here */
</style>
Step 6: Run Your Project
Finally, run your project to see the dynamic title in action:
npm run serve
Thank you for following along with this guide on setting up dynamic titles in your Vue.js application. I hope you found it helpful and straightforward.
Happy coding, and may your development journey be smooth and enjoyable!